In part 9 of this tutorial series we learned how to work with animations in Unreal Engine and we animated our Parasite enemy.
In this part of the tutorial we are going to code enemy AI behaviour and we are going to wrap up our game.
Creating The Enemy AI
Inside the BP_Parasite_Enemy Blueprint, create a new float variable, give it a name Wait Time, set the default value at 5 and check the Instance Editable checkbox:
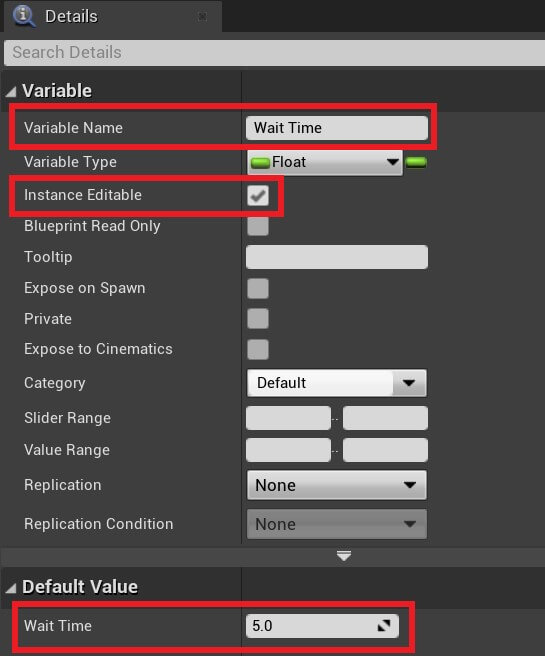
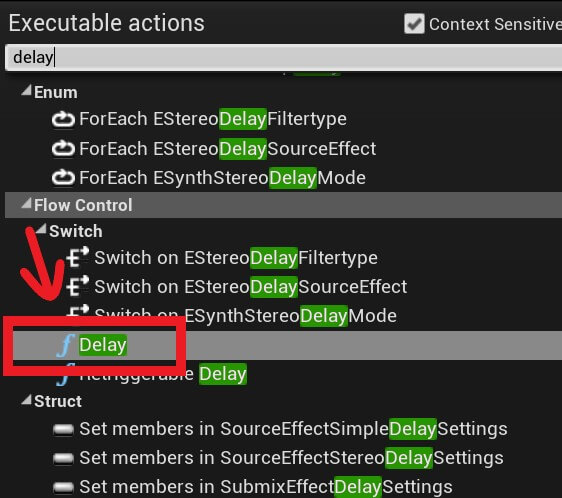
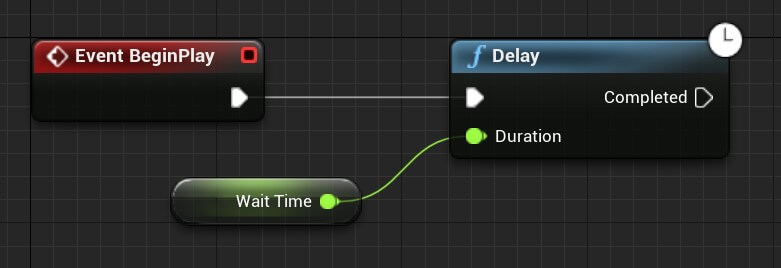
Don’t forget that we made that variable Instance Editable which means we can change the value of it on the instance of the Blueprint.
After we wait for the specified amount of time, we are going to call a new function that will move the enemy towards the player.
Inside the graph, Right Click and search for AI MoveTo function which is located under AI category:
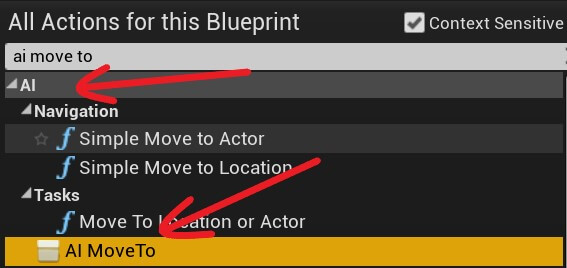
The AI MoveTo function takes a few paramaters. One of them is the Pawn parameter which is the Actor that will be moved with the AI MoveTo function which in our case is the Parasite enemy.
For that parameter we are going to get a reference to self by Right Click and search for self:
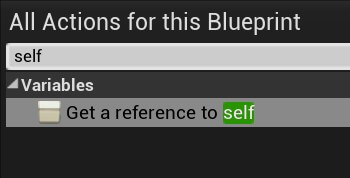
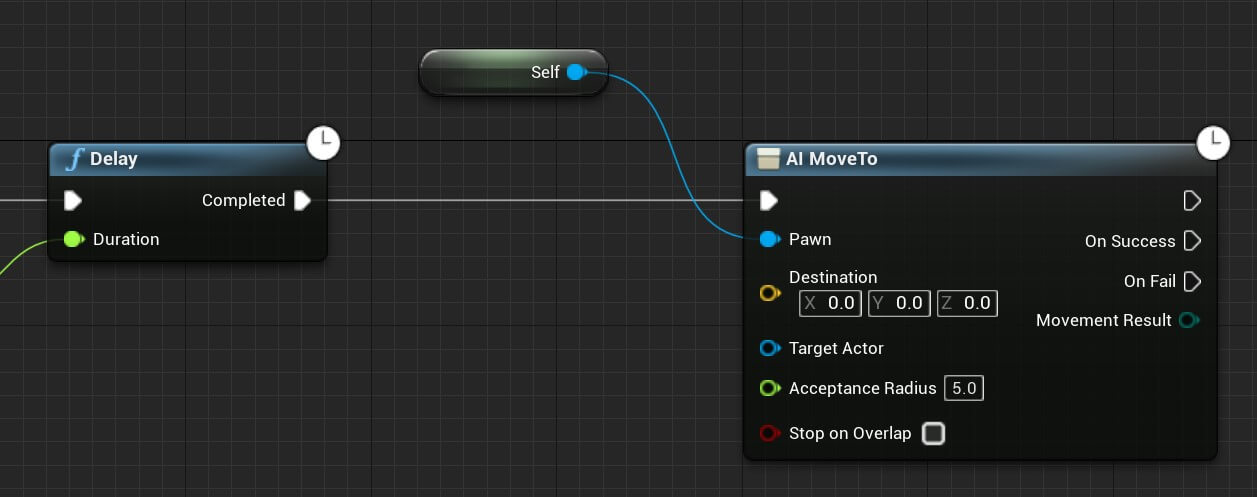
The next parameter that we need to provide is the Target Actor which will tell the AI MoveTo function where to go.
Since we are going to make the enemy follow our PlayerCharacter, we can get a reference to it by using Cast To, but since we already set up the BP_PlayerCharacter to be the default pawn, we can pass the returning value of the Get Player Character function to the Target Actor parameter:
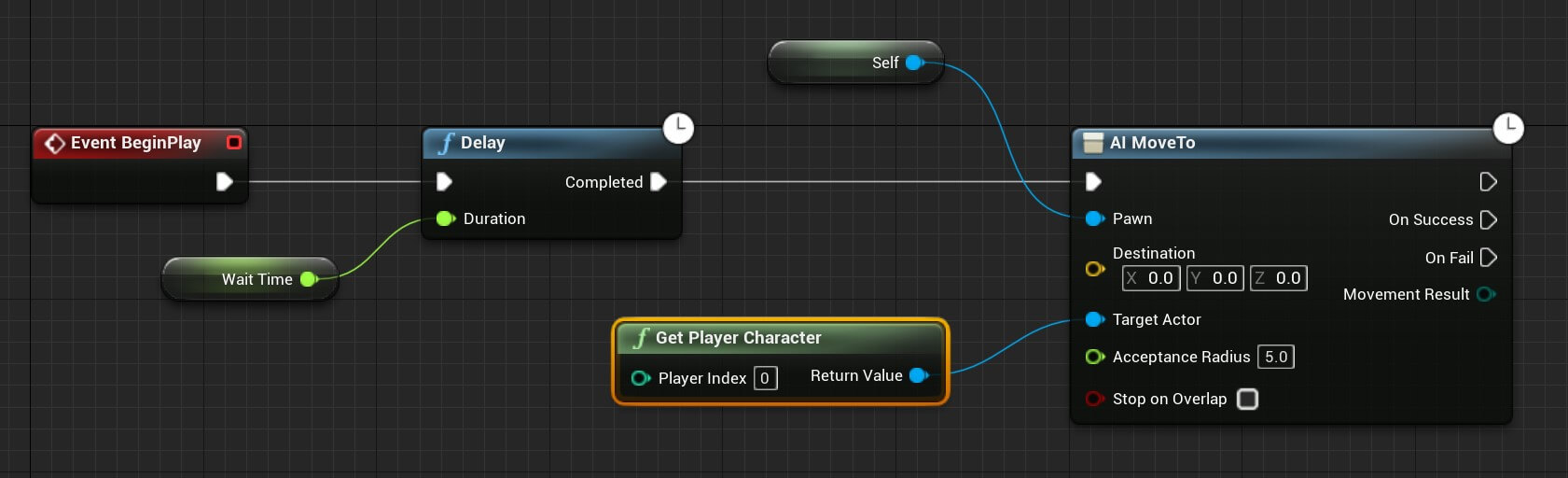
You can copy the nodes from here:
AI Nav Mesh Bounds Volume
The code for the AI behaviour is done, but before we can test it out we need to do one more thing.
In order for the AI to know where to move, we need to add something called Nav Mesh Bounds Volume.
Inside the level, in the Place Actors tab, click on All Classes and filter for nav mesh bounds volume:
- X = -117
- Y = 0
- Z = 57
- X = 106
- Y = 11
- Z = 4
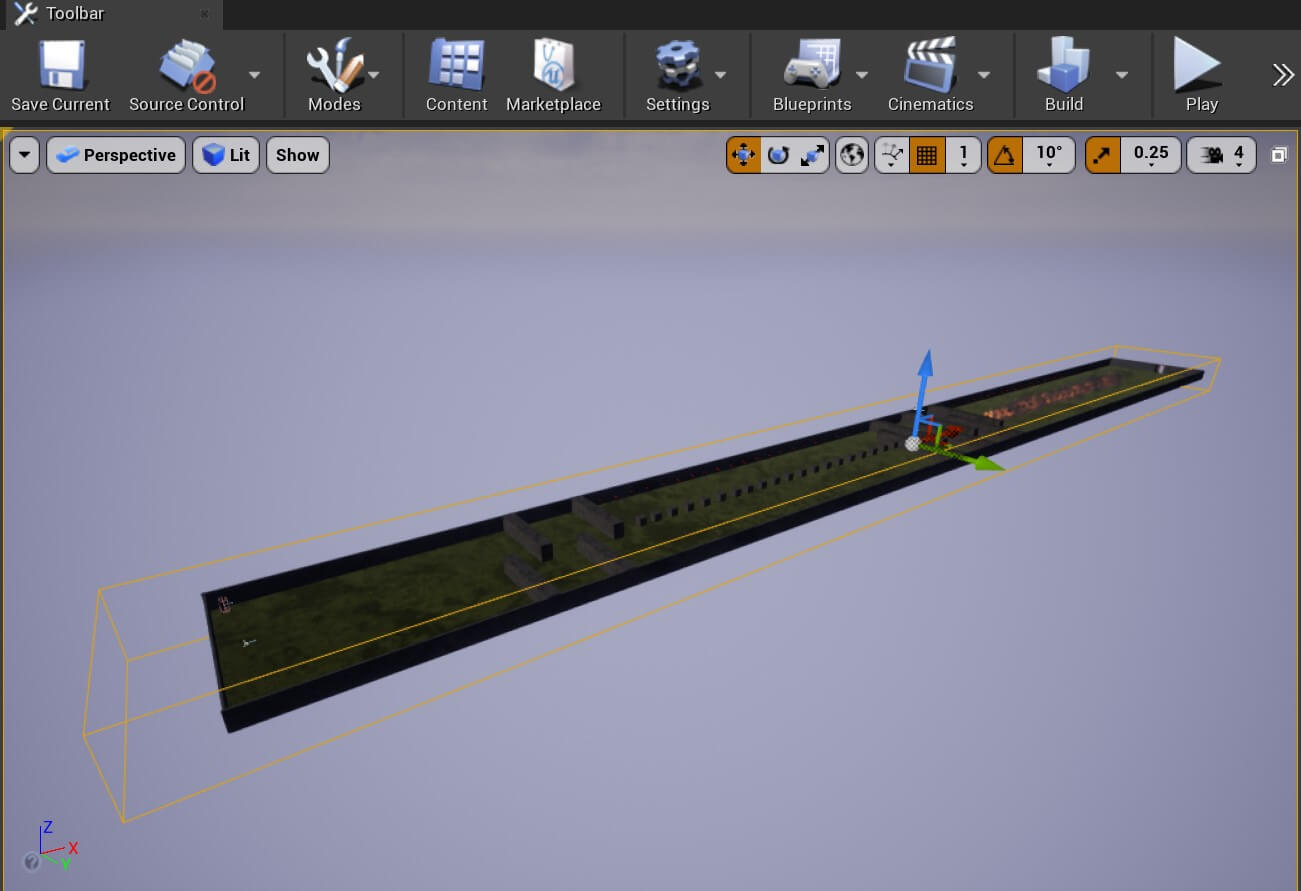
Connecting The Animation Blueprint With The Enemy Blueprint
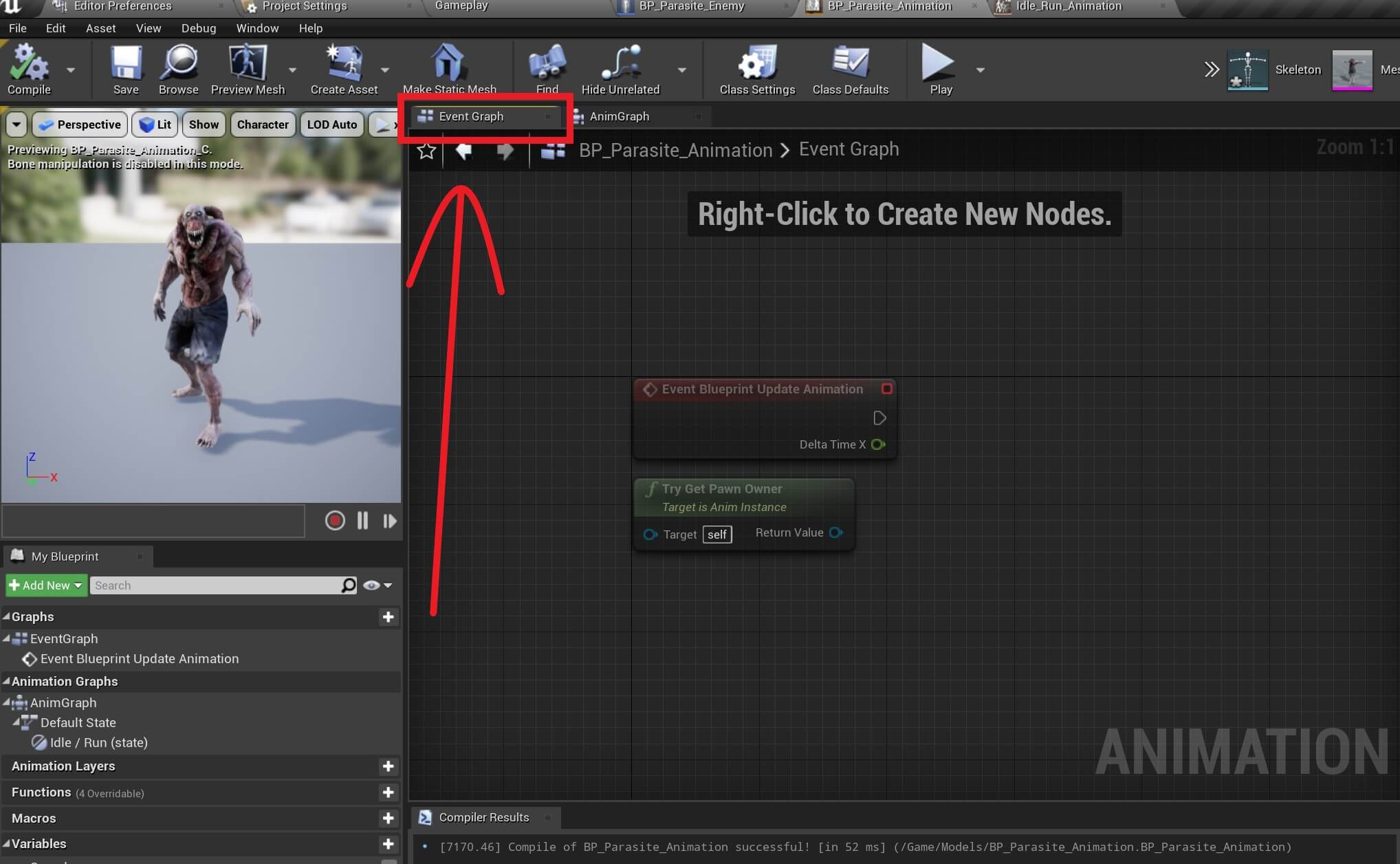
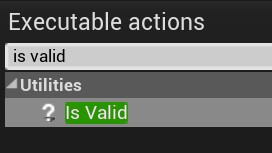
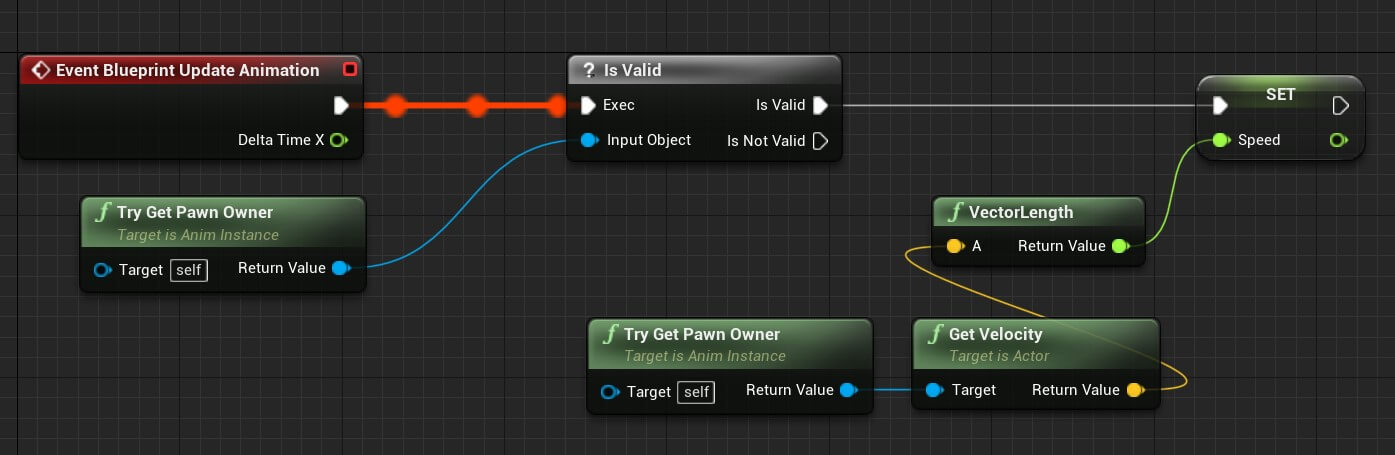
To set the value of the Speed variable we are using the velocity of the enemy. We get a reference to the enemy by calling the Try Get Pawn Owner function which again, will return the owner of the animation Blueprint which in our case is the Parasite enemy.
The velocity of an Actor increases when the Actor is moving, and the VectorLength will return the length of the velocity which is a float number.
The faster the enemy moves, the higher the value is for the length of the velocity.
When we test the game now, we will see that the running animation is being played when the enemy is moving:
As long as the enemy is moving the running animation is being played, and when the enemy reached its destination e.g. PlayerCharacter, the enemy stopped and the idle animation started playing.
Detecting Collision Between The Enemy And The Player
The last step in our game is to kill the PlayerCharacter when the enemy reaches him.
To do this, we need to add a new Box Collision component to the enemy.
Inside the BP_Parasite_Enemy, in the Components tab click on the Add Component button and filter for Box Collision:
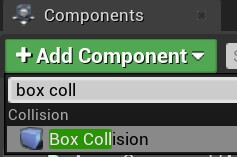
- X = 35
- Y = 32
- Z = 87
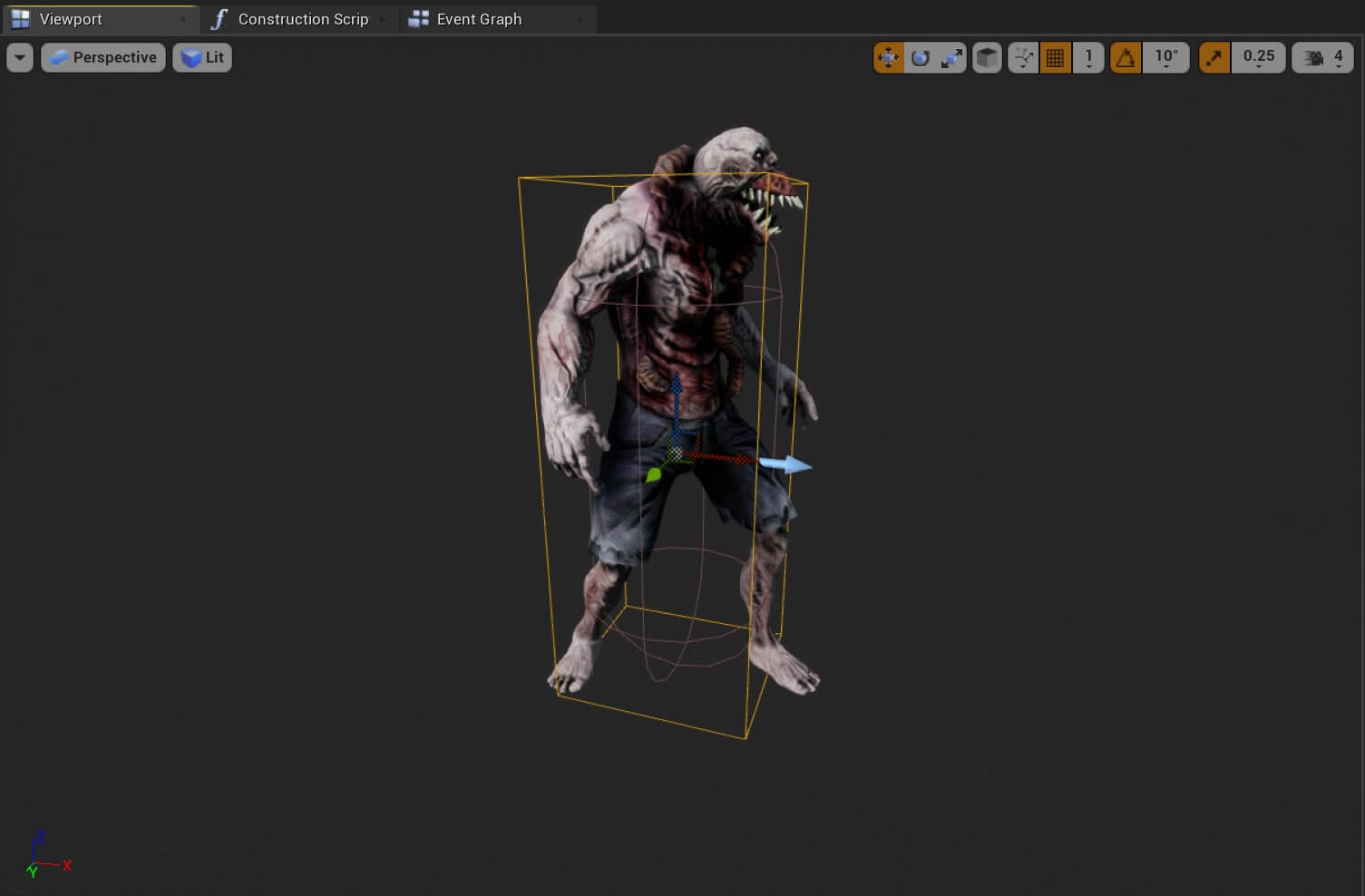
To detect collision in the code, we need to go inside the Event Graph, select the Collision Detection component and inside the Details tab scroll down to the Events settings and click on the green + button for the On Component Begin Overlap event:
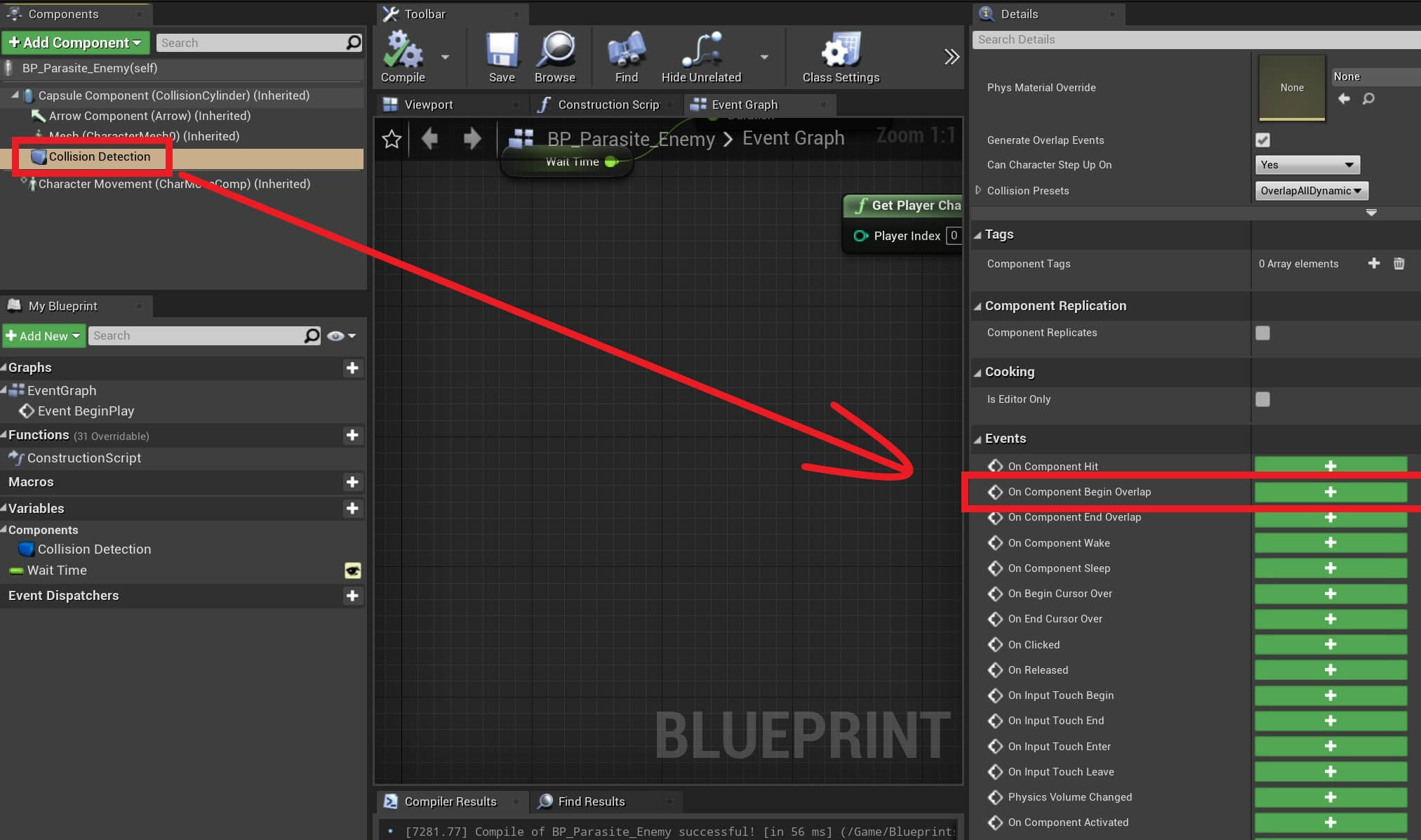

The same explanation we gave when we first introduced the collision detection between actors also applies in this situation, so if you need to refresh your knowledge you can click here.
Before we test the game, I am going to select the enemy in the World Outliner and in the Details tab change the Wait Time value to 2 so that we don’t have to wait for 5 seconds before the enemy starts moving:
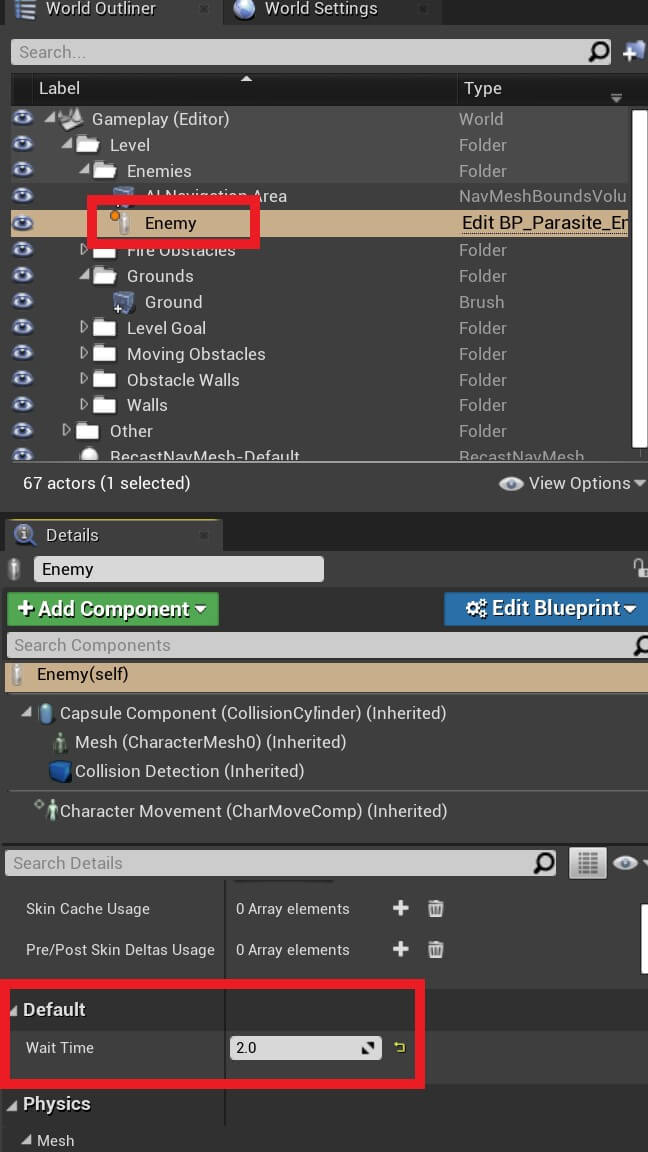
Now we can test the game:
2 thoughts on “Create A Parasite Platformer Game In Unreal Engine Part 10: Enemy AI Behaviour And Wrapping Up Our Game”
It was really helpful, and I enjoyied during following all of this tasts. Thanks!
Thank you so much for this! Great walkthrough of the entire process.