As soon as you start with Unity game development you will be bombarded with vectors. Most of the time you will use Vector2 for 2D games and Vector3 for 3D games.
So what are vectors and how can we use them?
Definition Of A Vector
Vectors are a fundamental mathematical concept which allows you to describe a direction and magnitude.
In math, a vector is pictured as a directed line segment, whose length is the magnitude of the vector and the arrow is indicating the direction where the vector is going:
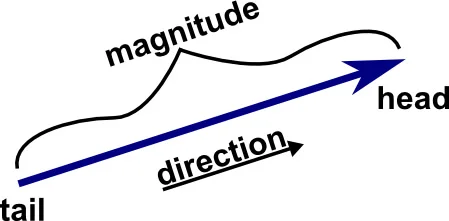
Vector2 And Vector3 In Unity
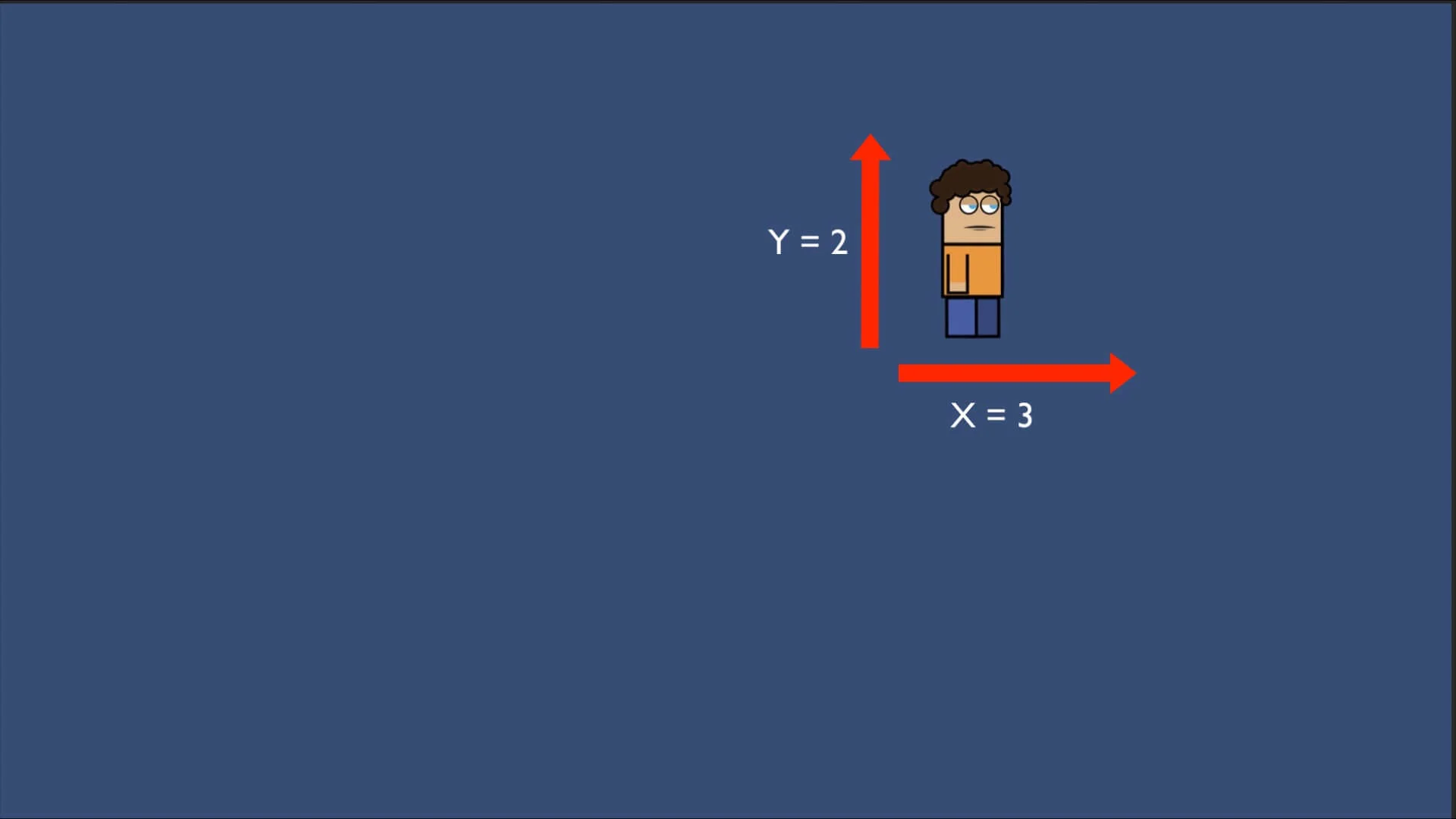
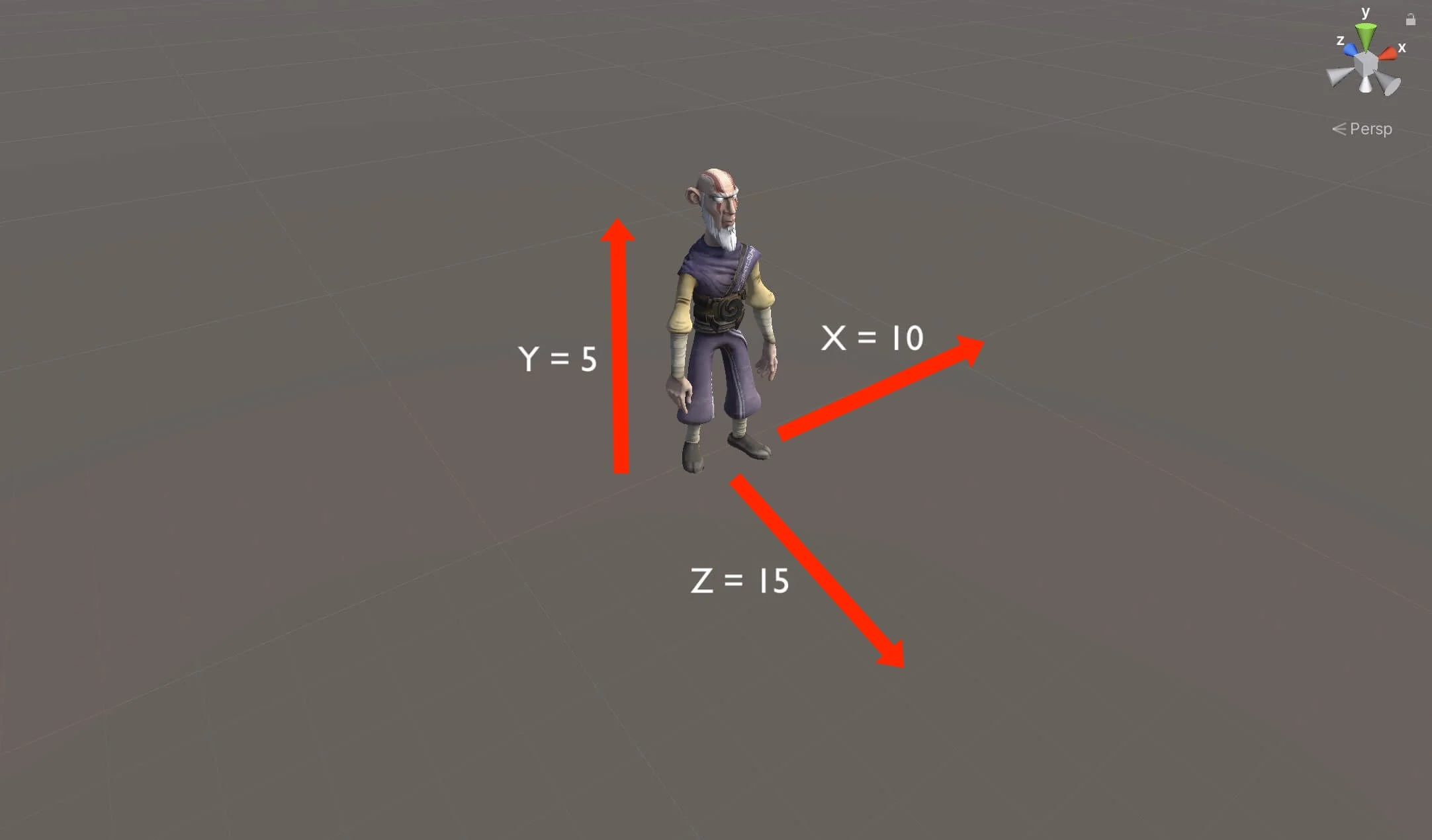
The values for the axis are located in the Position property of the Transform component of course:
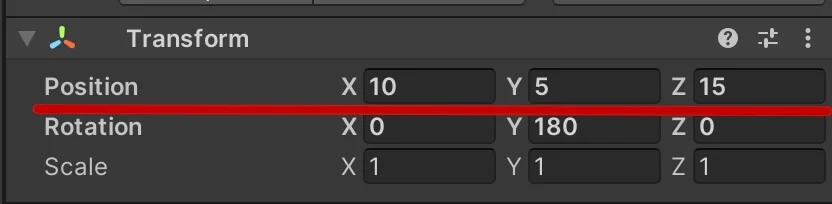
The Magnitude Of The Vector
One of the things we use vectors for is to know the magnitude of the game object.
But what is magnitude of a vector?
The magnitude is the distance between the vectors origin (0, 0, 0) and its end point. If we imagine a vector as a straight line, the magnitude is equal to its length as we saw in the first image:
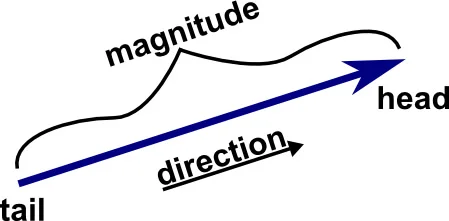
To calculate the magnitude in math, given a position vector → v = ⟨a,b⟩, the magnitude is found by magnitude = √a2 + b2 (square root of a squared + b squared).
In 3D it works the same way, except that we have one more axis in the calculation magnitude = √a2 + b2 + z2 (square root of a squared + b squared + z squared).
But in Unity we simply do this:
Vector3 testVector = new Vector3(10, 15, 20);
float testVectorMagnitude = testVector.magnitude;
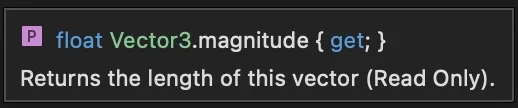
For What Do We Use The Magnitude Of A Vector
The magnitude of a vector is used to measure the speed of the vector. I say speed of the vector but its actually the speed of the game object.
For example, if we are moving the game object using its Transform component, we can limit the movement speed using the magnitude of the vector:
private float speedX, speedZ, moveSpeed = 10, maxSpeed = 100;
Vector3 tempVector;
void Update()
{
speedX = Input.GetAxisRaw("Horizontal");
speedZ = Input.GetAxisRaw("Vertical");
tempVector = transform.position;
// if the speed of the vector is less than the
// maximum allowed speed
if (tempVector.magnitude < maxSpeed)
{
tempVector += new Vector3(speedX, 0f, speedZ) * (moveSpeed * Time.deltaTime);
}
transform.position = tempVector;
}
rigidbody.velocity
rigidbody.velocity.magnitude
(Vector A - Vector B).magnitude
Squared Magnitude
float sqrtMagnitude = someVector.sqrMagnitude;
One thing that we need to be careful here is that this will calculate the magnitude without using the square root in the operation, which means it will return the magnitude squared e.g. the magnitude will 2x of its actual value.
So if we want to compare a distance between two vectors or the speed of the current vector we need to compare the squared values:
private Vector3 vectorA, vectorB;
private float maxDistance, maxSpeed;
float vectorA_Speed = vectorA.sqrMagnitude;
// multiple the max speed with itself
// to make it a squared value
float maxSpeedSquared = maxSpeed * maxSpeed;
// comparing the maximum speed
if (vectorA_Speed > maxSpeedSquared)
{
}
// calculating the distance between Vector A and B
float vectorAB_Distance = (vectorA - vectorB).sqrMagnitude;
// multiple the max distance with itself
// to make it a squared value
float maxDistanceSquared = maxDistance * maxDistance;
// comparing the distance between Vector A and B
if (vectorAB_Distance > maxDistanceSquared)
{
}
The Direction Of The Vector
One of the most common informations we need in a game is the direction of vectors which indicates in which direction a specific game object is going. This is used to move characters in the game, to create enemy AI and so on.
To get the direction of the vector we need to normalize it, and in Unity we normalize a vector like this:
private Vector3 vectorA;
Vector3 vectorA_Direction = vectorA.normalized;
// or
vectorA.Normalize();
Using normalized or Normalize will give us the direction of the given vector.
Now you are probably asking what is the difference between the two?
Both lines of code will normalize(return the direction) the given vector, but using normalized on a vector will return a new version of the same vector that we can store in a new variable, and the original version of the vector will stay the same.
Using the Normalize function however, will normalize the vector is self e.g. it will change the original vector.
If you hover over the normalized word in the code you will see the following explanation:

This means that the returned vector has a magnitude of 1 e.g. the length of that vector is 1, because this is how directions are represented in Unity.
For example:
// right direction
Vector3 right = new Vector3(1, 0, 0);
// left direction
Vector3 left = new Vector3(-1, 0, 0);
// up direction
Vector3 up = new Vector3(0, 1, 0);
// down direction
Vector3 down = new Vector3(0, -1, 0);
// forward direction
Vector3 forward = new Vector3(0, 0, 1);
// backward direction
Vector3 backward = new Vector3(0, 0, -1);
Depending on the axis, adding 1 or -1 value will point to a certain direction. This is connect to Unity’s coordinate system which looks like this:
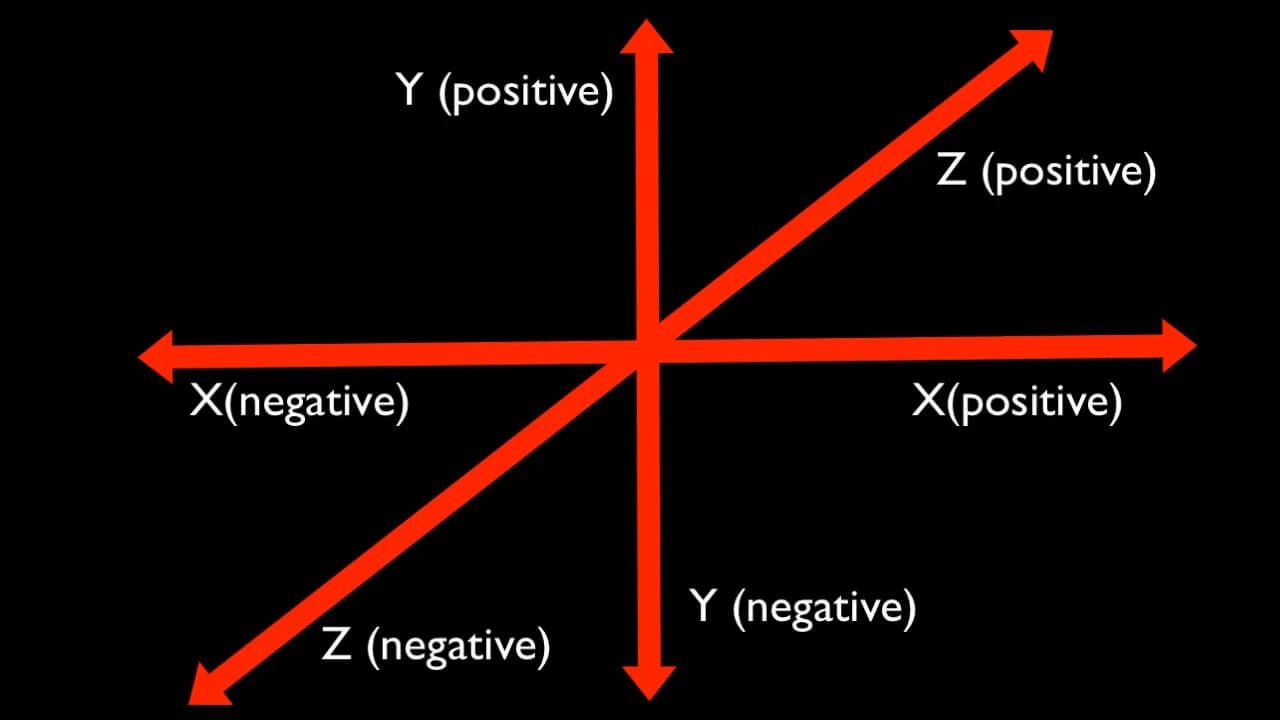
As you can see, X is positive on right side and negative on the left, Y is positive up and negative down, and Z is positive forward and negative backwards.
This is why you see the positive 1 and negative 1 values for the vectors in the code example above.
What Is The Difference Between Vector Magnitude And Vector Normalize
From all the examples we saw so far for the vector’s magnitude and normalization, we can conclude that there is a difference between them and they are both used for different purposes.
The magnitude returns a float, its a single dimensional value expressing vector length. It looses directional information but provides the length information which we can use to control the speed of the vector.
Normalization is a bit of an opposite operation – it returns vector direction, guaranteeing that the magnitude of the resulting vector is one. This means it looses the magnitude information but preserves the direction information which we can use to know where the game object is moving or to move a game object in a specific direction.
What Is The Use Of Normalized Vectors
private Vector3 vectorA, vectorB;
// calculate the direction
Vector3 movementDirection = (vectorB - vectorA).normalized;
// move vector A towards vector B by 0.5 unitys
vectorA += movementDirection * 0.5f;
Comments are closed.