Visual Studio Project Structure
When you open the project from the previous post, you will see some lines of code inside the project name.cpp file:
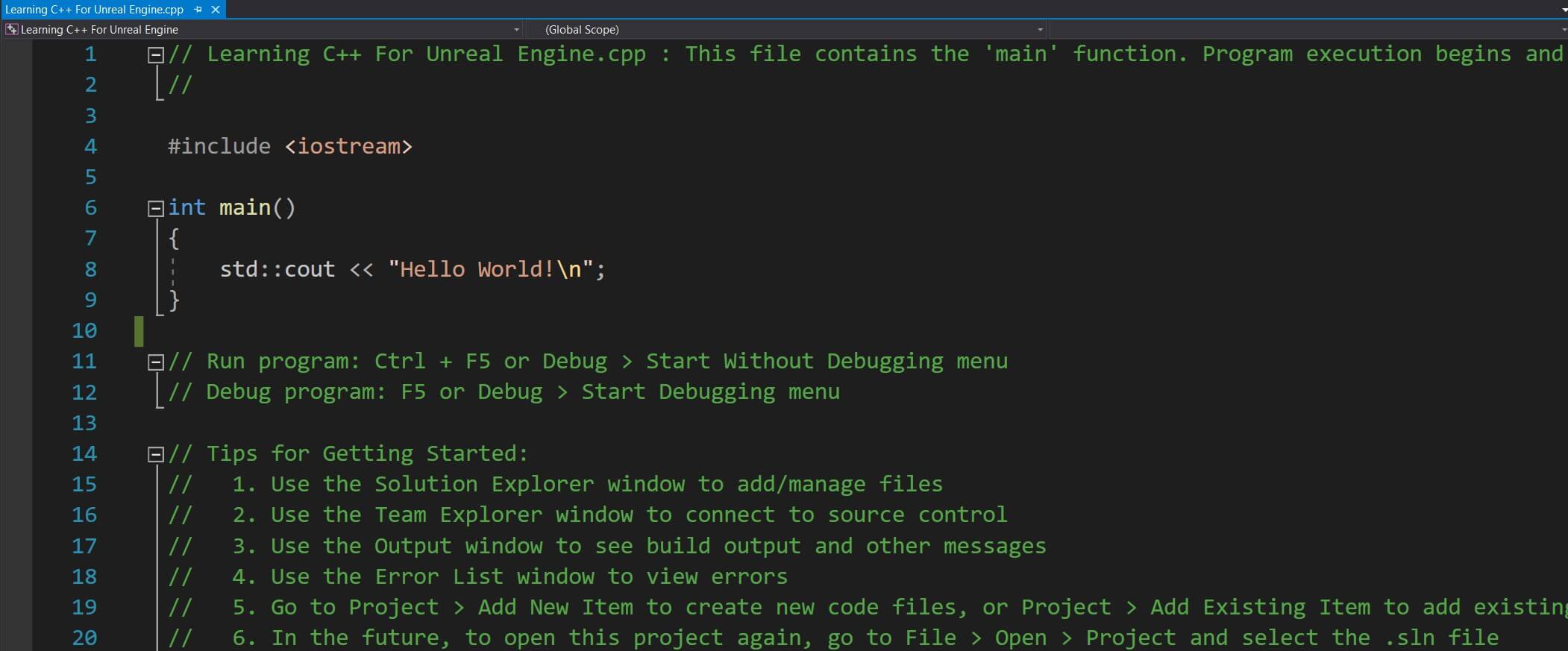
Every program needs a starting point e.g. where the program will start running.
Most programming languages have a function called main and that is where the program starts running because that function will be automatically called when the program runs, we will learn more about functions in upcoming lectures.
So the int main() {} that you see is the main function which will run our code.
The code that we want to run we need to put it between the curly brackets: { code to execute }, and it will be like this for every function, which we will see when we get to the lecture about functions.
The std::cout line that you see is used to print to the console of our program, and we will use that to print the values of variables and to demonstrate examples while we are learning how to code in C++.
And the sentences you see in green are comments. Comments are creating by using // and then you can write whatever you want to in your script file because comments are not executed by the compiler and they are used to document your code e.g. provide a brief description what your code is doing.
You can run this program by clicking on the Local Windows Debugger button:
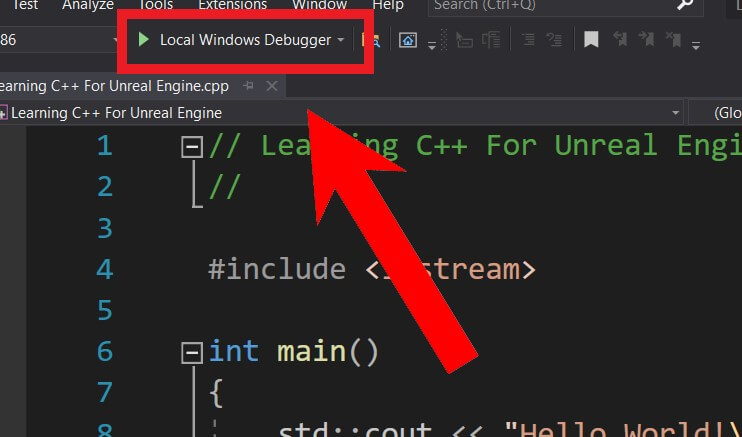
When the program executes a dark console will appear and the line std::cout << “Hello World!\n”; will print Hello World! to that console:
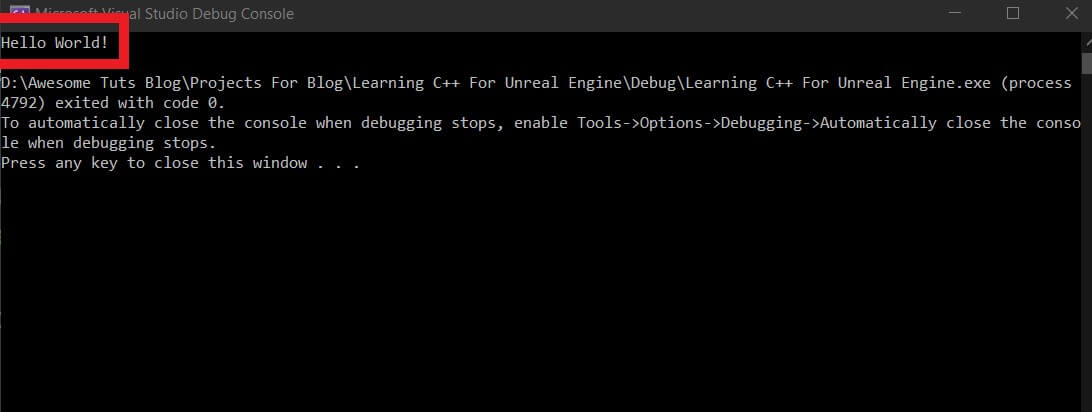
Variables - The Heart Of Every Program
The basis of every program, game and anything software related are variables.
Variables are used to represent every information that our game will have such as highscore, player progress, player health, enemy count, and everything else in between.
That being said, you can already assume that variables are numbers, which is true, there are number variables, there are character variables and there are boolean variables which can have a value of either true or false, and we will see all of that starting with number variables.
Whole Number Variables
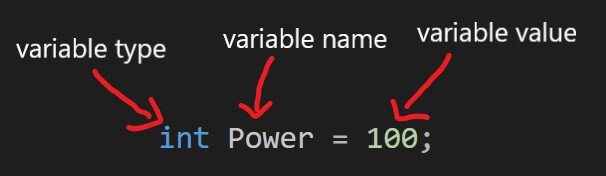
As you can see from the image, int is the variable type, it’s short for integer, Power is the name of the variable and this is totally up to us how we name our variables, but the name of the variable will always be meaningful like health, power, stamina, so that you know for what you are going to use that variable.
The number 100 after the equals sign is the value of the power variable and this is optional meaning you are not required to provide a value for the variable right away, you can assign the value later.
This means that we can also declare our power variable like this:
int Power;
In this case the value of the power variable is the default value for an integer which is 0, later on in the game you can always assign another value to the variable by typing:
Power = 100;
We will see a lot of examples of both ways when we start developing our games.
Decimal Point Numbers
Same as how we have whole numbers we also have decimal point numbers, and we have two types of decimal point numbers:
float Health = 2.3f;
double Mana = 3.1;
Both the float and the double represent decimal point numbers, and the difference in declaration is when you want to use a float you add f at the end of the value of the float when you declare it, which is what we did here when we set the value of the health variable to be 2.3 we also added f at the end.
For the double we don’t need to do that, it’s enough to just type the value that you want, after you declared the type of the variable to be a double of course.
Another difference between a double and a float is the precision, meaning up to how many decimal point numbers is the number 100% precise.
I didn’t research this in detail to know exactly up to which decimal point number a float and a double are precise, but I do know that a double is a little more precise than a float.
However, in game development, you will always use float instead of a double, and all the examples and projects that you will see and find online use a float instead of a double.
Character Variable
So far we saw number variables which have their purpose, but if you want to have character names in your game, or display an UI which has characters then you need a string.
This is how you declare a string:
string PlayerName = "Wizard";
The structure of the declaration is already known to us, string is the variable type, PlayerName is the name of that variable, and Wizard is the value of that variable.
What’s important to know is when you give a string variable a name you need to put the characters between quote marks ” your string goes here ” and anything you put between the quote marks will count as a string, even space.
So if you type
string PlayerName = "Kenny Florian";
that value is not the same as
string PlayerName = "KennyFlorian";
keep that in mind when you are creating your strings.
Boolean Variable
A boolean variable is a variable that can have one of the two values: true or false.
The reason for that is because in programming there is something called a control flow which allows us to take different actions depending on the condition at hand, that condition is either true or false and we use booleans for that.
This is how we declare a boolean:
bool IsDead = false;
bool is the variable type, and that is short for boolean. The rest of the
code you can figure out by yourself, IsDead is the name of the variable and its value is set to false.
This can also be true so if you change false to true it will also work.
Naming Conventions
Every programming language has it’s own naming conventions and these are general rules that you should follow while coding with that language.
As you saw so far, all starting characters of the names of our variables were capital, so it was Power, Health, Mana and so on.
And if the variable had two words in it’s name then the first letter of the first word and the first letter of the second word are capitalized, for example PlayerName or IsDead.
This is called camel case naming, and every programming language has this.
The point is that in C++ the names of the variables start with capital letter, then you use camel case if you have more words with every new word starting with a capital letter, these are the general rules.
There are always exceptions to rules of course, so even if you develop your own way of naming variables that is easy for you and your projects then you can use that.
The important thing is that your code is readable and when someone who looks at it for the first time can understand what your code is doing.
Some people might not agree with this, but seeing that every company has their own way of coding and their own style of writing code then there is no harm in doing this.
I personally sometimes name variables with first letter capital sometimes I don’t, so you will see Health and health in my code, or playerHealth and PlayerHealth.
Sometimes it’s much easier to add an underscore in the variable name to differentiate it more easily, and still make your code readable and understandable.
The naming convention applies to functions as well, but for functions every time the first letter is capitalized, for example Attack or DealDamage and so on, and we will see this when we start creating functions don’t worry.
For now this is enough, we introduced all the variables that we will be using through our development and we will see a lot of examples of manipulating variables for various purposes.
Comments are closed.