In the lecture about variables we learned that we have three types of number variables, two are decimal point variables and one is a whole number variable.
It’s only logical to think that you can perform mathematical operations with those variables, and you are correct.
The Scariest Part Of Programming, Well, Not So Much
The fact is that you only need to know basic operations like addition, subtraction, multiplication and division.
If you can perform those basic math operations on numbers from 1 to 100, you know more than enough math to learn how to code.
Not convinced?
Let’s take a look at some some examples.
Addition
Inside the main function in your script write the following lines of code:
float Health = 50.0f;
float HealValue = 2.0f;
std::cout << "The remaining health is: " << Health + HealValue;
Run the program and you will see this printed in the console output:
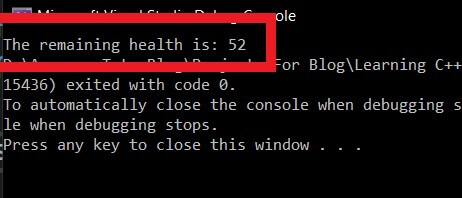
And there you go. It’s as simple as adding two numbers together.
We can also create a third variable and store the calculated numbers inside that variable:
float Health = 50.0f;
float HealValue = 2.0f;
float NewHealth = Health + HealValue;
std::cout << "NewHealth Value Is " << NewHealth;
If we print this to the console you will see the same output from the previous example.
One thing to note is that we are using floats for current calculation, the same way we can use doubles or integers.
We can add the following lines of code:
int a = 2;
int b = 3;
int sum = a + b;
std::cout << "The sum of a and b is: " << sum;
When you run this application you will see “The sum of a and b is: 5” is printed in the console output.
Subtraction
int a = 2;
int b = 3;
int subtraction = a - b;
std::cout << "The result is: " << subtraction;
If you run the application you will see that the result that is printed is 1.
The result of a subtraction can also be negative, which means if we change the values of a and b:
int a = 5;
int b = 10;
int subtraction = a - b;
std::cout << "The result is: " << subtraction;
Multiplication
int a = 5;
int b = 10;
int multiplication = a * b;
std::cout << "The result is: " << multiplication;
When you run the app you will see the result is 50. You can also multiply negative numbers:
int a = -5;
int b = 10;
int multiplication = a * b;
std::cout << "The result is: " << multiplication;
Division
The last operation is division. If we change the values of a and b to:
int a = 10;
int b = 5;
int division = a / b;
std::cout << "The result is: " << division;
When you run the app you will see the result is 2.
The same rules with negative numbers apply for division as well. In fact, the same rules apply to all operations that we saw so far.
One thing that you need to keep in mind when using division is, depending on your needs you will use different types of numbers.
What do I mean by this?
Let’s take a look at this example:
int a = 5;
int b = 2;
int division = a / b;
std::cout << "The result is: " << division;
Casting? What Is That?
int a = 5;
int b = 2;
float division = (float)a / (float)b;
std::cout << "The result is: " << division;
float division = a / b;
The result would still be 2, because you didn’t specify that you want the variables a and b to be treated as floats, so they will be treated as integers even though the result variable division is a float.
Comments are closed.