In the previous post we talked about pointers and we saw how powerful they are because they allow us to access the variable from its memory address.
References and pointers are very similar, and we will use them a lot in our game development journey.
Declaring A Reference
When we talked about pointers we saw that a pointer points to the memory address of the variable.
A reference on the other hand points to the variable directly.
What does that mean?
Let’s take a look at an example:
int a = 5;
int& refTo_a = a;
std::cout << "The value of a is: " << a << "\n" ;
std::cout << "\nThe value of refTo_a is: " << refTo_a << "\n";
When declaring a reference we use the ” & ” character after the variable type. Since we want to have a reference to an integer, the reference variable is also going to be an integer.
Because we want a reference to the variable itself, we simply assign to the reference variable ” a ” and don’t use any special character along with it as we did with pointers.
When you run the code above you will see this printed in the console:
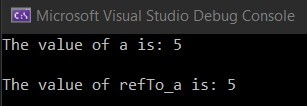
As you can see the value of both variables is 5.
We can also get the memory address of both variables:
std::cout << "The memory address of a is: " << &a << "\n" ;
std::cout << "\nThe memory address of refTo_a is: " << &refTo_a << "\n";
When you run this code you will see this in the console(of course the memory numbers will be different for your app but both numbers should be the same):
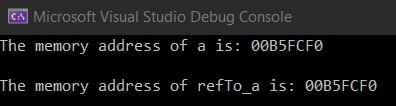
Both variables have the same memory address because the reference variable is pointing to the ” a ” variable.
From what we see so far, pointers and references are doing the exact same thing.
Difference Between Pointers And References
So what is the difference between them, if any?
To understand the difference between pointers and references let’s take a look at an example:
int a = 5;
int* pointerTo_a = &a;
std::cout << "The value of a is: " << a << "\n" ;
std::cout << "The value of pointerTo_a is: " << *pointerTo_a << "\n";
int b = 34;
pointerTo_a = &b;
std::cout << "\nThe value of a is: " << a << "\n";
std::cout << "The value of pointerTo_a is: " << *pointerTo_a << "\n";
Here we have an integer variable and a pointer to that variable. We first print the value and pointer value for variable ” a “, then we change the pointer from variable ” a ” to variable ” b “, and again we print the value for variable ” a ” and the current pointer value:
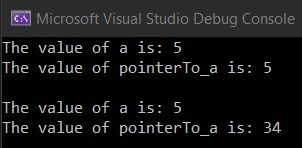
As you can see from the print, the value of ” a ” doesn’t change when we set the pointerTo_a = &b, but pointerTo_a changes from 5 to 34.
This means that when you change the pointer value of a certain variable, the original variable is going to stay the same.
What will happen if we do the same thing with references?
Let’s take a look:
int a = 5;
int& refTo_a = a;
std::cout << "The value of a is: " << a << "\n" ;
std::cout << "The value of pointerTo_a is: " << refTo_a << "\n";
int b = 34;
refTo_a = b;
std::cout << "\nThe value of a is: " << a << "\n";
std::cout << "The value of pointerTo_a is: " << refTo_a << "\n";
Repeating the same scenario but this time with references we have variable ” a ” and a reference to that variable refTo_a.
First we print values for variable ” a ” and refTo_A and then we change refTo_a to ” b ” and we print the same values again:
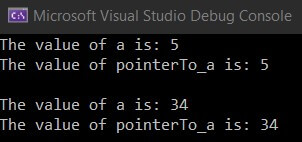
Now you will notice that the first print prints the value 5 for both variable ” a ” and refTo_a, but when we change refTo_a = b, now the second print prints 34 for both variable ” a ” and refTo_a.
Because refTo_a is a reference to variable ” a “, changing the value of refTo_a also changes the value of variable ” a ” even though we didn’t modify ” a ” directly.
Translating that into a visual example it would look like this:
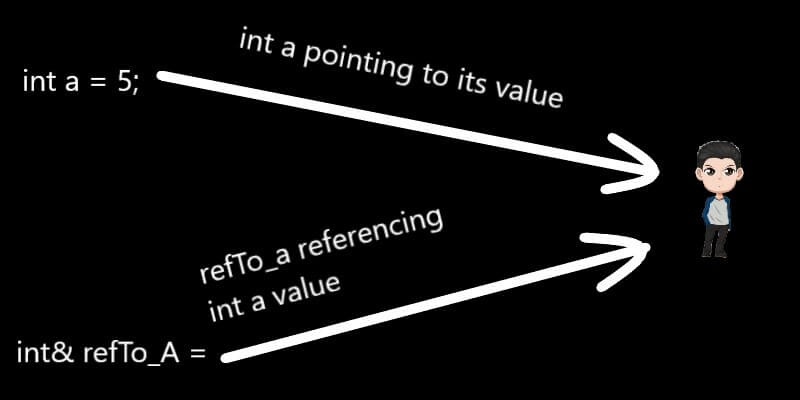
Whereas with pointers, if you change the value of pointerTo_a the value of ” a ” stays intact because the pointer is now pointing to a new memory address which has nothing to do with variable ” a “.
Where To Go From Here
In this post you learned how to use reference to manipulate with variables.
To continue with your learning journey you can take a look at our Classes And Objects Lecture which is the next lecture in this tutorial series.