Variables are the foundation of programming. To represent anything in your game, be that player’s name, health status, how much ammo you have left and so on, you need variables.
As you can see from the few examples I mentioned what we can represent with variables, you already know that these variables are numbers and characters.
Creating Our First Script
Before we start to write any code, let us create our first script. In the project tab Right Click -> Create -> C# Script:
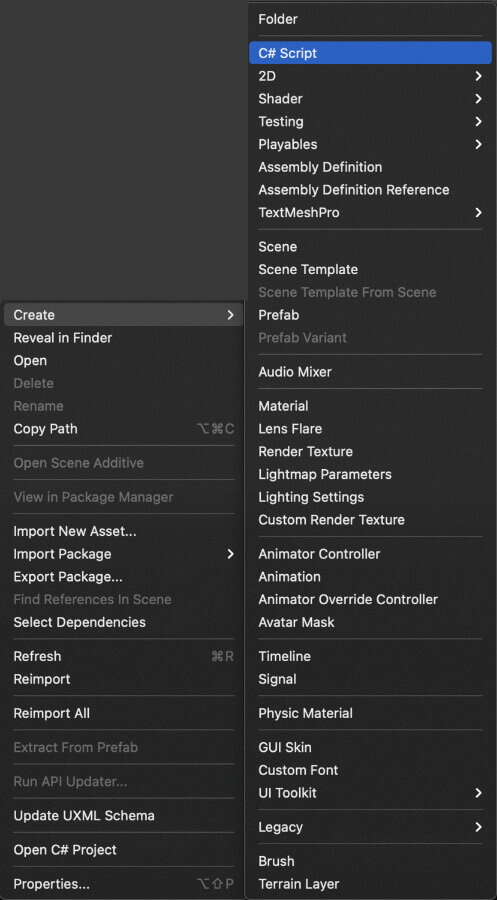
As soon as you create a new script in Unity, you will be prompted to give it a name. Give this script a name: LearningHowToProgram
The name itself is not important for our lectures that will come in these posts, but later when you start creating your game you will give meaningful names for your scripts.
These names represent the task for which you want to use the script such as PlayerMovement, PlayerAnimation, EnemAI and so on.
Double click the script and this will open it in Visual Studio:
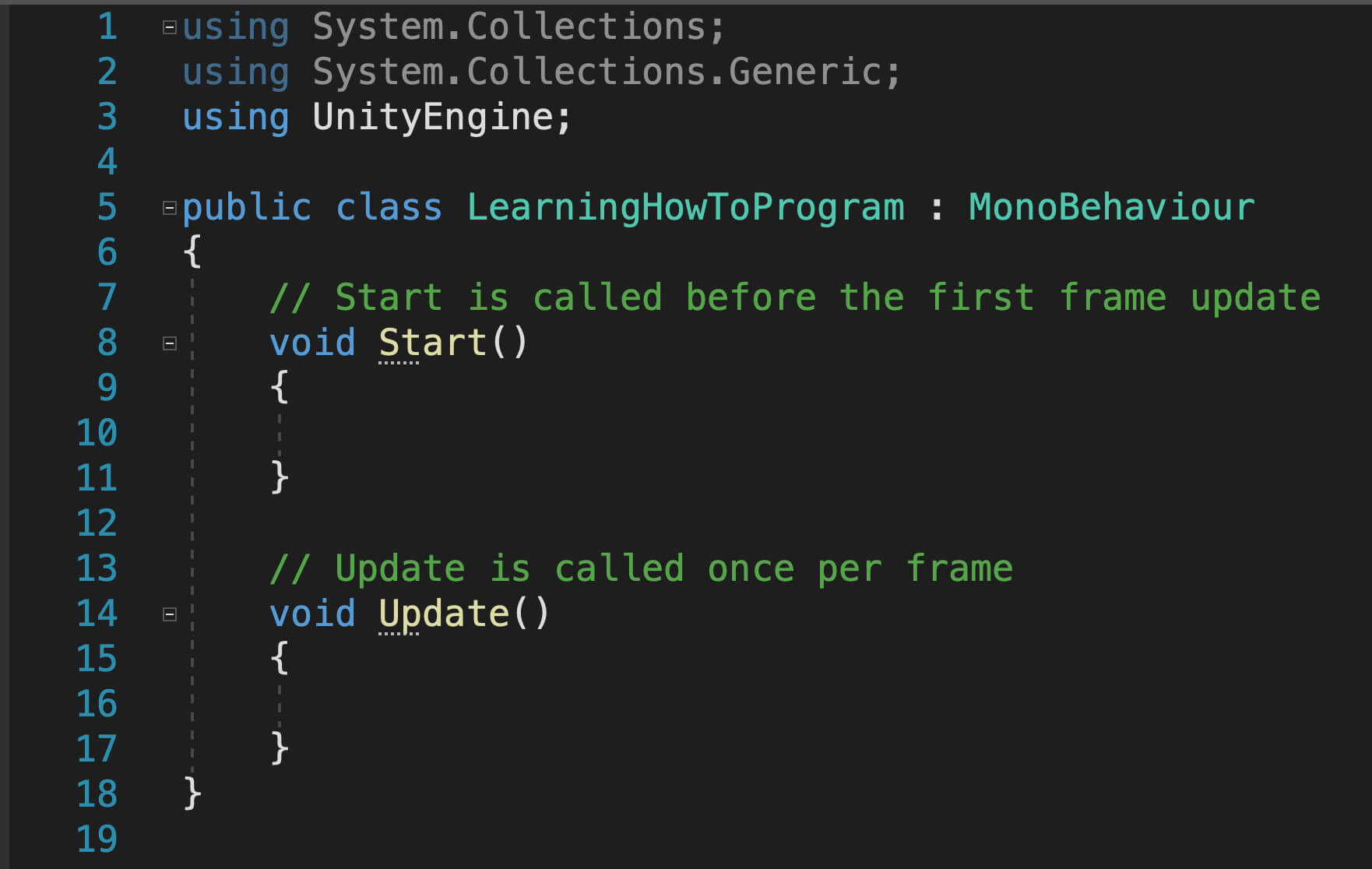
We are not going to go through every part of the script to explain what everything represents, but what’s important for us at the moment to understand is that the Start function that you see is used for initialization of your script.
For example, if you want to calculate some values before your game starts, then you would do that in the Start function. Later down the road we will discuss the initialization functions in more depth, because there are in total 3 functions that serve this purpose.
The reason why I mentioned Start now is because any code that we want to print to the console as an example, we will type it in the start function. Now we can jump into the juicy parts and finally introduce variables.
Decimal Point Variables
As we already mentioned variables are numbers and characters, but there are multiple types of variables that we can use, such as whole numbers or decimal point numbers. So the first variable that I will introduce to you is called a float:
float heath = 100f;
Type this line of code above the Start function so that you have something like this:
float health = 100f;
void Start()
{
}
All variables that we want to use inside the specific script we will declare above the Start function or to be more precise right below the public class declaration.
We will see more examples of that, but now let’s talk about the structure of declaring a variable and break down the line of code that we wrote.
When declaring a variable, we first give it a type, then we give it a name, and optionally we give it a value, same as what we did with the float example:
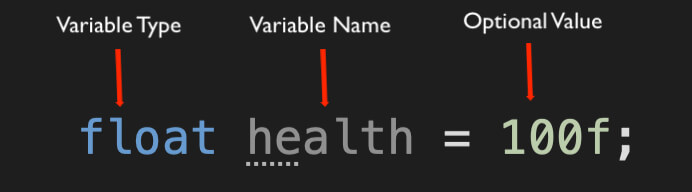
The variable type and name are mandatory when you want to declare a variable of any type, but the value is optional which means that you don’t have to give your variable a value right away, you can calculate it later during gameplay.
The variable that we declared in this example is called a float, and it represents a decimal point number.
Now you will notice that the number we gave our float as its value is 100, it’s not 100.0 which represents a decimal point number, and the reason for that is when you have 0 after the decimal point you can omit it and still the number will count as a decimal point number in the code.
The compiler will add .0 at the end of that number when it’s ready to use it and you don’t have to do any extra work. If we were to declare 100.5 then we would have to specifically add .5 at the end of the number.
The “f” suffix at the end of the declaration is used to denote that this number is a float type number. This is because we have another variable type that also represents decimal point numbers which is called a double, and this is how we declare it:
double power = 100;
In this example you see that we gave our double a value of 100, but we didn’t add f at the end. The compiler automatically knows that this number is a double because we specified that with the variable type, and we don’t need to add f at the end.
Same as with the float, you can omit the .0 at the end, but if you have any other value other than 0 as a decimal point number then you must type it.
Now the question arises, what is the difference between a float and a double? Because both variables represent decimal point values, so what is the difference, if any.
From all the research that I did, I came to the conclusion that the main difference between the two variables is that the double is more precise when it comes to the decimal point numbers.
To give a rough example, a float can be 100% precise up to the 15th decimal number e.g. 2.435353535353535 and a double can be 100% precise up to the 25th decimal point number e.g. 2.4353535353535353535353535.
These examples are rough examples as I already mentioned, or better yet, this is me trying to use human language to explain the difference between the two variables as best as I can, but for the majority of our development we will use floats to represent decimal point numbers in our projects.
I’ve been using Unity for 7 years and not once have I used a double to represent a decimal point number.
Whole Number Variables
Moving forward, the same way how we have decimal point numbers, we also have whole numbers and they are called integers. This is how we declare an integer variable:
int speed = 10;
int is short for integer, and that represents the variable type, then we give it a name and after that the optional value. Integers don’t have any letters that you can add at the end of the variable declaration so we just give it a value we want and that’s it.
Character Variables
Next we have a string variable which is a character type variable, and this is how we declare it:
string playerName = "Knight";
string is the type of variable, so we declare it first, same as with the other variable types, then we give it a name and an optional value.
One thing to note is that whenever you want to type a string value, you do that in quotes, so any value that you want to type needs to be in between the “” marks.
It’s important to know that anything you type between the quote marks will be counted as a string variable, so if you type space, this counts as a character, which means that
string playerName = "Carl Johnson";
is not the same variable as
string playerName = "CarlJohnson";
so keep that in mind.
Boolean Variables
The last variable that we have is called a boolean, and this a variable that can only have true or false as its values. This is how we declare it:
bool isAlive = true;
bool is the type of the variable, isAlive is the name and true is the value. We can also type false instead of true, but these are the only two values that the boolean variable can have.
This is because booleans are used in conditional statements that can only be true or false, and we will see many examples of this.
Naming Conventions For Variables
Another thing to note is when using C# and Unity you will use a camel case way of writing variable names, such as isAlive.
The name of the variable starts with a lower case letter then every beginning letter of every new word in the variable name is capitalized such as Alive in this case.
The are also other naming conventions that you can follow such as using underscores and giving your variable a name like this:
bool _isAlive = true;
Or even
bool is_Alive = true;
We will use both of these examples in our development so you will get used to it.
Conclusion
After you declared all of these variables this is how your script should look like:
float health = 100f;
double power = 100;
int speed = 10;
string playerName = "Knight";
bool isAlive = true;
void Start()
{
}
This would be it when it comes to variable types that we can use to develop our games and these 5 types of variables are the foundation of any game that you will create.
From now on we will use all of these variable types A LOT in our development so you will become a master variable manipulator 🙂
One Last Tip - Comments
You probably noticed the words above the Update and Start functions when you created your script:
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
}
These are called comments and they are not counted as lines of code.
This means that you can write anything you want in the comment and they are primarily used to document code.
You create comments by typing // and after that you can add notes that will help you understand what your code is about when you come back to it after a few months.
One thing to note is that don’t overdo the comments and write paragraphs about what your code does, instead use few words that describe what your code is doing. For example:
// players movement
// detecting enemy presence
// dealing damage
And so on.
Where To Go From Here
In this lecture you learned about the most essential part of every game that we will create – variables.
To continue your learning journey you can take a look at our Math Operations With Variables Lecture which is the next lecture in this tutorial series.
1 thought on “C# Programming In Unity – Introduction To Variables”