One of the things that we will do a lot in Unity game development is having a so-called Singleton class that will control the workflow of our game.
With the workflow of the game I mean keeping track of the players progress, how many enemies are in the game, unlocking power ups and so on.
And this is best done with a static class.
What Is A Static Class
The main difference between a normal class and a static class is that we can’t create objects from a static class.
We can still have variables and functions inside the static class, but we need to use the keyword static when we are declaring them.
And now you are probably wondering, if we can’t create objects from a static class, how can we access the variables and functions declared in a static class?
Well, it’s very simple, by using the class name.
How To Declare A Static Class
public static class GameplayController
{
}
And this is how we denote that a class is a static class.
Now when it comes to variables and functions, we declare them the same way we would declare them in a normal class, but we add the static keyword:
public static class GameplayController
{
public static int levelNumber;
public static void LoadNewLevel()
{
Debug.Log("A new level is being loaded");
}
}
The static keyword goes after you declare the accessibility modifier or the variable or the function.
To access the variables and functions of a static class, simply use the name of the static class name.variable name or static class name.function name.
Inside the Start function of the LearningHowToProgram class write the following lines of code:
void Start()
{
GameplayController.levelNumber = 2;
Debug.Log("Level number is: " + GameplayController.levelNumber);
GameplayController.LoadNewLevel();
}
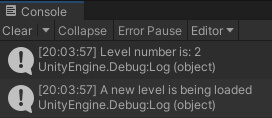
And this would be pretty much it when it comes to static class.
We only used one variable and one function as an example to demonstrate how a static class works, but you can create all other variable types as well, just make sure to add the static keyword.
The same goes for functions. You can create functions that return a value, functions that take parameters and so on.
We will see a lot of examples of static classes in our development as they are used a lot in Unity game engine.
Comments are closed.