Now that we understand what are classes and how to use them, we are going to introduce a new concept which is called inheritance.
The first question that comes up is what is inheritance?
Well, inheritance is the ability to inherit a class and have access to all the variables and functions the inherited class has.
In order to understand this, make sure that the Player class is defined like this:
public class Player : MonoBehaviour
{
private int health = 100;
private string playerName;
public void Attack()
{
Debug.Log("The player is attacking");
}
public void SetHealth(int healthValue)
{
health = healthValue;
}
public int GetHealth()
{
return health;
}
public void SetName(string newName)
{
playerName = newName;
}
public string GetName()
{
return playerName;
}
}
public class Warrior : Player
{
}
Instead of MonoBehaviour we are going to type Player, and this tells the class to inherit from the Player class.
When you create any class by default it will inherit from MonoBehaviour, we can change that and set the class to inherit any other class that we want, in this case the Player class.
Inside the editor on the Player game object remove the Player script by clicking on the 3 dots of the script component and then press Remove Component:
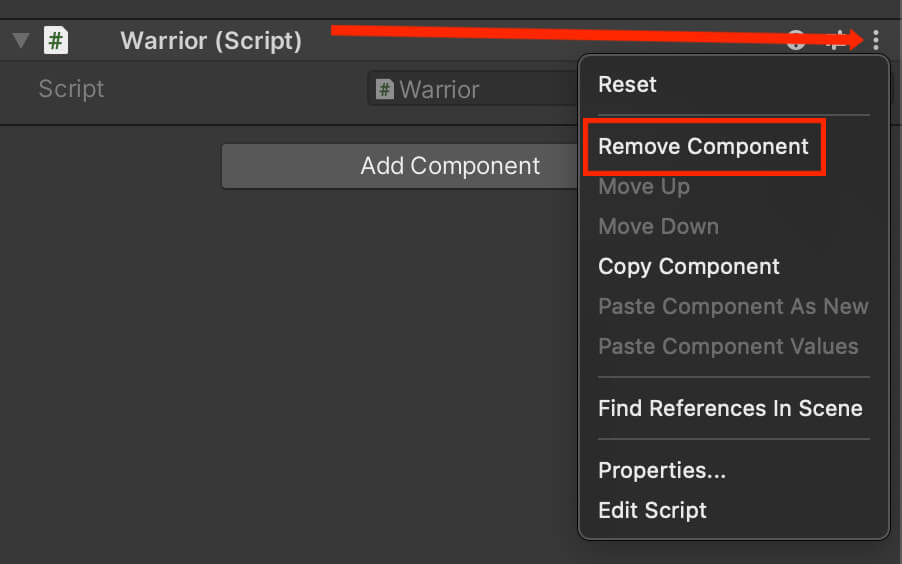
After that attach the new Warrior script on the game object. Modify the code inside the LearningHowToProgram class:
private Warrior warrior;
void Start()
{
warrior = GameObject.Find("Player").GetComponent();
warrior.SetName("Warrior");
Debug.Log("The name of our character is: " + warrior.GetName());
}
When you run the game this is what you will see in the Console:
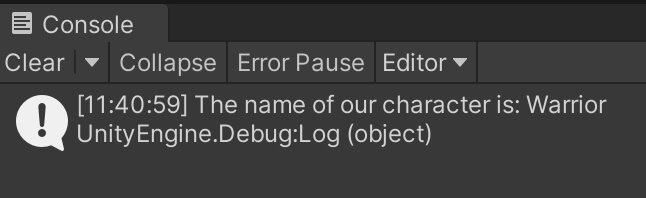
Now you are wondering if I am some sort of wizard and how did I manage to get an empty class to have functionalities. Well, that is the power of inheritance.
Even thought the SetName function is inside the Player class, since we inherited that class in the Warrior class we can use the functions and variables from the Player class.
When I say we can use variables and functions from the inherited class I mean we can use public variables and functions declared inside the inherited class which is also called the parent class.
For example the health variable is set to private inside the Player class, if we to access the health variable directly from the Warrior class like this:
warrior.health = 20;
We will get the famous Player.health is inaccessible due to its protection level:
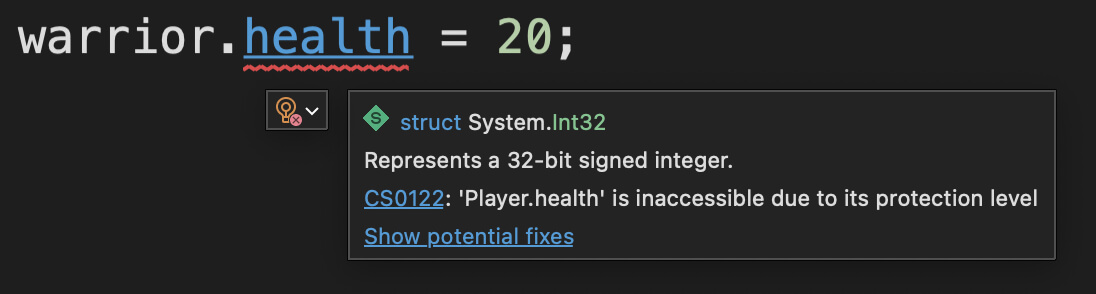
Overriding Functions
The Player class has a function called Attack which is defined like this:
public void Attack()
{
Debug.Log("The player is attacking");
}
We can use this function from the Warrior class by calling:
warrior.Attack();
If we run the game this is what we will see in the Console:
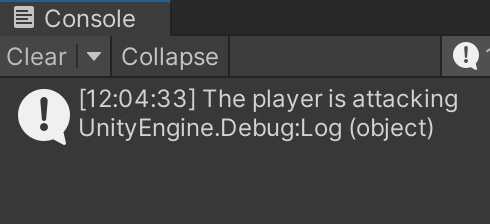
This is too generic especially for a video game.
We created a new class Warrior but when we use the inherited Attack function from the Player class it prints “The player is attacking”.
We want to create our own version of the Attack function inside the Warrior class so that we can modify the function to suit the Warrior class. For this we use a concept called overriding.
In order to mark the Attack function as overridable we need to define it like this:
public virtual void Attack()
{
Debug.Log("The player is attacking");
}
public override void Attack()
{
Debug.Log("The warrior is attacking with an axe");
}
With the keyword “override” we are denoting that we are changing the functionality of the Attack function inside the Warrior class and we will provide the new functionality with our own code.
So now if we type:
warrior.Attack();
After we run the game this is what we will see inside the Console:
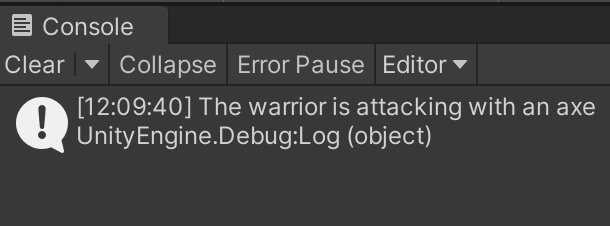
Before we implemented the override functionality we saw that Player is attacking was printed in the console, but now our modified code has printed “The warrior is attacking with an axe” in the Console.
The Benefits Of Inheritance
This concept is very useful because it allows us to create classes that will have a common behaviour for all object types that we plan to create out of that class.
One of the examples of this is if we have a game where we have multiple characters that can walk and attack.
We can model a base class that has the behaviour of walking and attacking and then in every child class where we inherit the main class we can modify the movement and attack to suit the specified character.
That way one character can attack by throwing fire, another character can attack with ice, some other character can shoot bullets and so on, but they are all using one and the same function inherited from the parent class.
This was the last part in our introduction to programming with C# in Unity. Now we are equipped with enough knowledge to start creating real world games and see in action every concept that we covered so far.
3 thoughts on “C# Programming With Unity – Inheritance”
In case this is helpful to someone, there is an error on this line :
warrior = GameObject.Find(“Player”).GetComponent();
It should be:
warrior = GameObject.Find(“Player”).GetComponent();
Seem like the comment field is bugged and removed some words, let me try again.
It should be:
warrior = GameObject.Find(“Player”).GetComponent();
Seems like it can’t be typed but the GetComponent part is missing the syntax