Functions are used to group a block of code.
This can be useful in many ways, for example, in the lecture about operations with variables we performed addition on two variables, what if you need to do that often in your code, it would be very tedious to type a + b every single time you need to calculate a new value.
This is where functions come into play.
How Do We Declare A Function?
We already saw examples of two functions as soon as we created our script LearningHowToProgram – Start and Update. These functions are inherited from MonoBehavior that’s why we saw them in the script. We will talk about inheritance later.
To declare a function we first type void which means that the function doesn’t return a value, we will explain this in a moment, then we give a function it’s name, then we open and close parentheses (), and open and close curly brackets {}:
void CalculateTwoNumbers()
{
// code that you want to execute
}
Any code that you want this function to execute you will place between {} as you can see from the example above. Using the example from the lecture about operations with variables, we can rewrite the code in a function:
void CalculateTwoNumbers()
{
float a = 1.5f;
float b = 2.2f;
float c = a + b;
Debug.Log("The value of c is: " + c);
}
You will declare this function anywhere between the { } of the class itself:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class LearningHowToProgram : MonoBehaviour
{ // class declaration starts here
void Start()
{
}
void CalculateTwoNumbers()
{
float a = 1.5f;
float b = 2.2f;
float c = a + b;
Debug.Log("The value of c is: " + c);
}
} // class declaration ends here
And in order to call the function to execute the code that’s inside we simply call it by it’s name:
CalculateTwoNumbers();
Put the line of code above in the Start function and run your game. This is what you will see in the Console:
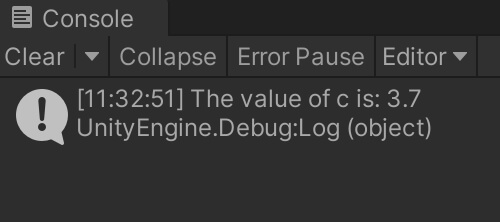
This is the same result you would see if you place the same lines of code from CalculateTwoNumbers function inside the Start function directly.
But the main difference is that you don’t need to type all those lines of code every single time, instead you can call the CalculateTwoNumbers function as many times as you want and every type it will print the same value.
Functions With Parameters
The function we wrote is not exactly a useful one because it will always calculate the value of the same two numbers and print out their value.
There is a better way how we can rewrite this function so that it will calculate any two numbers we want and it goes like this:
void CalculateTwoNumbers(float a, float b)
{
Debug.Log("The value is: " + (a + b));
}
We added two floats a and b between () of the function.
Anything that we add between () of a function is called a parameters and now our CalculateTwoNumbers function takes two parameters which will be used to perform the calculation.
Another thing that you see is that we calculated a + b directly inside of Debug.Log instead of creating another float value to act as a sum of a and b.
Note that we are performing the calculation of a and b inside additional parenthesis so that our game will know that a + b is a mathematical operation that it must perform instead of adding those two numbers to the string on it’s left side.
Now when we call the CalculateTwoNumbers function we need to provide two float parameters inside the () and they must be separated by comma same as how they are separated in the function declaration:
CalculateTwoNumbers(2.9f, 5.5f);
Put the line of code above in the Start function and run the game. This is what you will see in the Console:
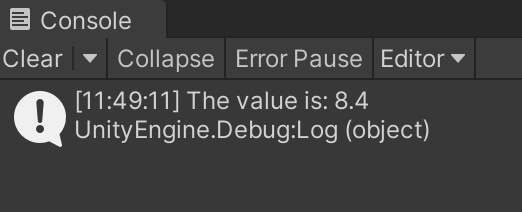
And this will work with any two numbers that you provide as parameters to the function.
What is important to know is that you are not limited by the number of parameters that you can have in a function. For this example we used 2 floats, but you can have 3 floats, or 5 floats and so on.
Also, you are not limited by one variable type as a parameter, so you can have 2 floats and 2 integers as parameters, or 2 floats and 2 strings, or 1 string, 1 float and 1 integer and so on.
I highly encourage you to experiment by changing the types of parameters on the function and printing them out to see how everything works.
Function That Return A Value
float CalculateTwoNumbers()
{
return 2.2f + 5.4f;
}
Debug.Log("The value is: " + CalculateTwoNumbers());
When you run the game you will see this printed in the Console:
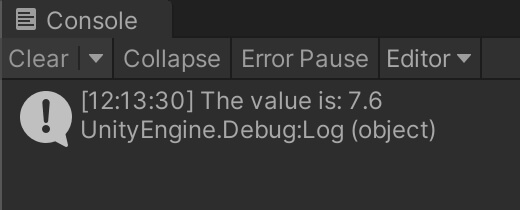
We can also create a new float variable and “capture” the returning value inside of it like:
float c = CalculateTwoNumbers();
Debug.Log("The value is: " + c);
When you run the game you will see that the value printed in the Console is the same like the one we had in the previous example. All of this would work the same way for other types of parameters like strings, integers or booleans.
Function That Takes Parameters And Returns A Value
The previous function we wrote only returns a sum of 2.2 and 5.4 which is not practical in development. We can rewrite our CalculateTwoNumbers function to take parameters and then return a sum of the provided parameters when we call the function:
float CalculateTwoNumbers(float a, float b)
{
return a + b;
}
Now we can provide any two numbers as parameters and have this function calculate and return the sum of the two numbers:
Debug.Log("The value is: " + CalculateTwoNumbers(1.1f, 6.7f));
When you run your game now this is what you will see in the Console:
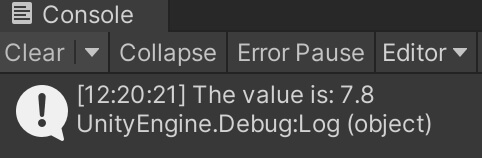
Conclusion
In programming you will have multiple blocks of code that will represent some logic that your game uses to run.
That block of code can have 10, 100, 300 or more lines of code, and instead writing all those lines of code every single time we need it, we can simply group it in a function and then call the function name and it will execute all those lines.
This way it’s much easier to write and group or code to make it more readable and performance friendly.
As we progress more and start creating games we will see examples of functions very often, but for now I highly encourage you to experiment with the examples we introduced in this post.
Try changing the parameter types and use Debug.Log to print the result in the Console. This is the best way to learn.
Where To Go From Here
In this lecture you learned how to reuse your code by grouping it into functions. This also makes your code cleaner and more optimized.
To continue your learning journey you can take a look at our Conditional Statements Lecture which is the next lecture in this tutorial series.
Comments are closed.